Interactive dashboards are powerful tools for visualizing data, making it easier to analyze and make informed decisions. By combining the strengths of Vue.js and D3.js, developers can create dynamic and responsive dashboards that are not only visually appealing but also highly functional. This guide will walk you through building interactive dashboards using Vue.js and D3.js, providing a comprehensive understanding of how to leverage these technologies effectively.
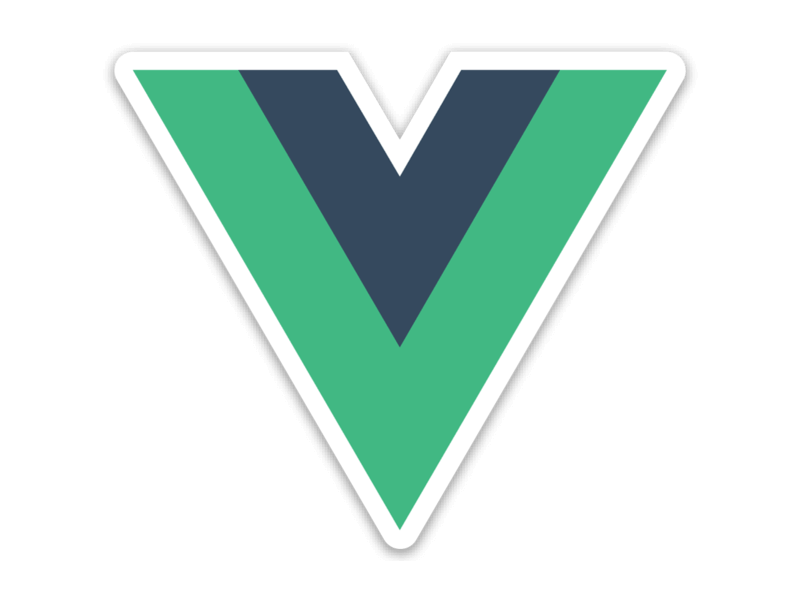
1. Introduction to Vue.js and D3.js
Vue.js is a progressive JavaScript framework used for building user interfaces. It offers a reactive and component-based approach that simplifies the development of complex web applications. Vue.js is known for its simplicity, flexibility, and ease of integration with other libraries.
D3.js (Data-Driven Documents) is a powerful JavaScript library for creating data visualizations. It uses web standards like SVG, HTML, and CSS to bring data to life. D3.js is highly flexible and allows developers to create custom visualizations, making it ideal for crafting interactive dashboards.
Combining Vue.js and D3.js allows you to build interactive dashboards with a structured framework for application logic and the robust capabilities of D3.js for data visualization.
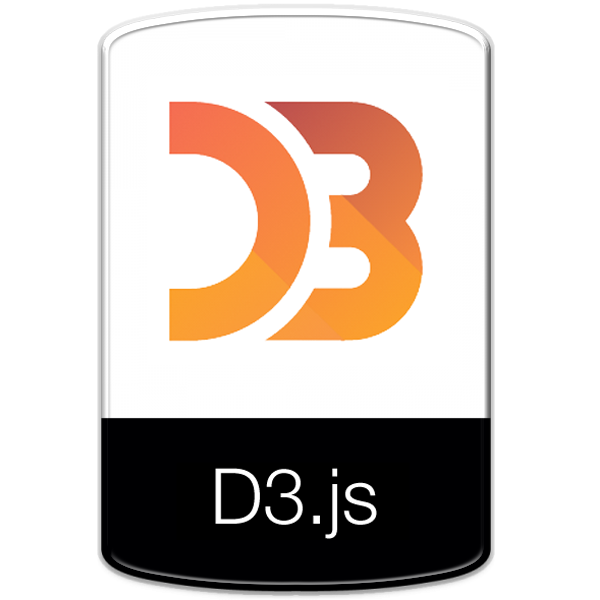
2. Setting Up Your Development Environment
Before we begin building our interactive dashboard, you need to set up your development environment. Here are the steps to get started:
Prerequisites:
- Node.js installed on your machine
- Basic understanding of JavaScript, Vue.js, and D3.js
Step 1: Initialize a Vue.js Project
- Open your terminal and create a new Vue.js project using Vue CLI:
vue create interactive-dashboard
- Navigate to your project directory
cd interactive-dashboard
Step 2: Install D3.js
- Inside your project, install D3.js via npm
npm install d3
With Vue.js and D3.js installed, your environment is now ready for development.
3. Building the Dashboard Structure
The first step in building the dashboard is to structure the application using Vue components. This approach keeps the code modular and manageable.
Step 1: Create the Dashboard Layout
- In your
src/components
directory, create a new file namedDashboard.vue
. - Define the basic layout for the dashboard
<template>
<div class="dashboard">
<h1>Interactive Dashboard</h1>
<div class="charts-container">
<!-- Components for charts will go here -->
</div>
</div>
</template>
<script>
export default {
name: 'Dashboard',
};
</script>
<style scoped>
.dashboard {
padding: 20px;
}
.charts-container {
display: flex;
flex-wrap: wrap;
gap: 20px;
}
</style>
Step 2: Add to Main App
- Import and include the
Dashboard.vue
component in your mainApp.vue
<template>
<div id="app">
<Dashboard />
</div>
</template>
<script>
import Dashboard from './components/Dashboard.vue';
export default {
name: 'App',
components: {
Dashboard,
},
};
</script>
4. Integrating D3.js for Data Visualization
Now that the basic structure is set up, let’s integrate D3.js to create interactive charts.
Step 1: Creating a Simple Bar Chart Component
- Inside the
components
directory, create a new file namedBarChart.vue
. - Implement the bar chart using D3.js
<template>
<div ref="chart"></div>
</template>
<script>
import * as d3 from 'd3';
export default {
name: 'BarChart',
props: ['data'],
mounted() {
this.createChart();
},
methods: {
createChart() {
const data = this.data;
const width = 500;
const height = 300;
const margin = { top: 20, right: 30, bottom: 40, left: 40 };
const svg = d3.select(this.$refs.chart)
.append('svg')
.attr('width', width)
.attr('height', height)
.append('g')
.attr('transform', `translate(${margin.left},${margin.top})`);
const x = d3.scaleBand()
.domain(data.map(d => d.name))
.range([0, width - margin.left - margin.right])
.padding(0.1);
const y = d3.scaleLinear()
.domain([0, d3.max(data, d => d.value)])
.nice()
.range([height - margin.top - margin.bottom, 0]);
svg.append('g')
.selectAll('rect')
.data(data)
.join('rect')
.attr('x', d => x(d.name))
.attr('y', d => y(d.value))
.attr('height', d => y(0) - y(d.value))
.attr('width', x.bandwidth())
.attr('fill', 'steelblue');
svg.append('g')
.call(d3.axisLeft(y));
svg.append('g')
.attr('transform', `translate(0,${height - margin.top - margin.bottom})`)
.call(d3.axisBottom(x));
},
},
};
</script>
<style scoped>
div {
width: 100%;
display: flex;
justify-content: center;
align-items: center;
}
</style>
Step 2: Using the Bar Chart in the Dashboard
- Import and include the
BarChart
component inDashboard.vue
<template>
<div class="dashboard">
<h1>Interactive Dashboard</h1>
<div class="charts-container">
<BarChart :data="chartData" />
</div>
</div>
</template>
<script>
import BarChart from './BarChart.vue';
export default {
name: 'Dashboard',
components: {
BarChart,
},
data() {
return {
chartData: [
{ name: 'A', value: 30 },
{ name: 'B', value: 80 },
{ name: 'C', value: 45 },
{ name: 'D', value: 60 },
{ name: 'E', value: 20 },
{ name: 'F', value: 90 },
{ name: 'G', value: 55 },
],
};
},
};
</script>
<style scoped>
.dashboard {
padding: 20px;
}
.charts-container {
display: flex;
flex-wrap: wrap;
gap: 20px;
}
</style>
5. Enhancing Interactivity and Adding More Visualizations
To create a fully interactive dashboard, consider adding more visualization components like line charts, pie charts, or maps. Each component can leverage D3.js for rendering and can respond to user interactions such as clicks, hover effects, and zooming.
Here are some enhancements you can make:
- Tooltips: Add tooltips to display data values when hovering over chart elements.
- Filters and Controls: Implement dropdowns or sliders to filter data dynamically.
- Transitions and Animations: Use D3.js transitions to animate data changes smoothly.
6. Performance Optimization Tips
When building dashboards with multiple visualizations, performance can be a concern. Here are some tips to keep your application performant:
- Lazy Loading: Load heavy data or charts only when needed.
- Efficient DOM Manipulation: Use Vue’s reactive system wisely to minimize unnecessary DOM updates.
- Data Management: Use Vuex or a similar state management library to handle data efficiently.
Conclusion: Transforming Data into Insights with Emperor Brains
Interactive dashboards are essential for transforming raw data into actionable insights. By combining the power of Vue.js and D3.js, developers can build feature-rich dashboards that are both visually appealing and highly interactive. Whether you’re tracking business metrics, analyzing scientific data, or visualizing financial performance, these tools provide the flexibility and control needed to craft bespoke solutions.
At Emperor Brains, we specialize in creating dynamic web solutions tailored to your needs. Our expertise in modern JavaScript frameworks, including Vue.js and D3.js, allows us to build interactive dashboards that not only look great but also perform exceptionally. If you’re looking to harness the power of data visualization for your business, explore our services at https://emperorbrains.com/.
Ready to take your data visualization to the next level? Connect with Emperor Brains today and let’s bring your data to life!
2 Comments
This is a fantastic guide! Interactive dashboards are game-changers in data analysis. Can’t wait to implement these insights into our projects! 💡
Great